Integrated Payment Solutions
For businesses looking to easily integrate robust payment solutions into their software applications
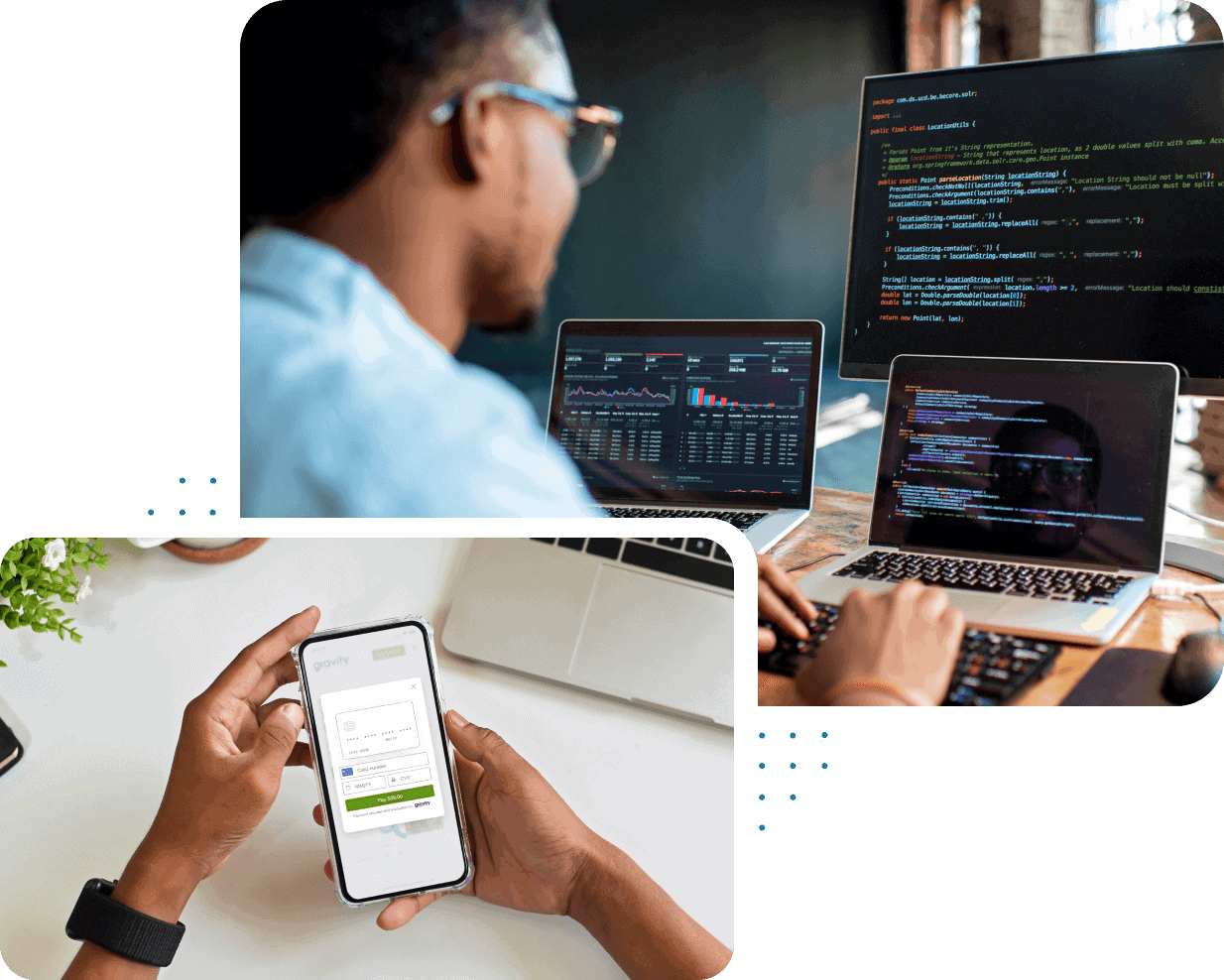
In-Store
Save time, reduce errors, and enjoy centralized in-software reporting.
Online
Sell goods, collect deposits, and take recurring payments through our unified online payment system.
On-the-Go
Collect payment anywhere using portable and virtual terminals that run on 4G.Ā
Integration Methods
Intuitive payments integration from our fast and scalable cloud-based solution – emergepay.
Modal
Our simple form is a reactive, mobile-friendly UI solution with fixed size and placement for easy implementation.
Embedded Form (URL Page)
Our instant solution provides a pre-built form within an iFrame
Custom Form
Our custom form (Hosted Fields) is a fully customizable solution that gives you design and data flexibility.
Checkout
Our eCommerce solution for developers seeking complete control over the flow of a transaction while remaining out of scope for PCI.
Digital Wallets
Our solutions are compatible with Google Pay and Apple Pay digital wallet services.
Direct Payments (ACH)
Recurring payments through a secure web environment using clientsā bank accounts and routing numbers.
//transactionType: "CreditSale"
//install the npm modules: 'npm install express cors emergepay-sdk'
var express = require("express");
var cors = require("cors");
var sdk = require("emergepay-sdk");
//Your private and secure creditials
var oid = "your_oid"; //Organization ID
var authToken = "your_authToken"; //Secretly generated specific to you org
var environmentUrl = "environment_url"; //Sandbox or Production environment
var emergepay = new sdk.emergepaySdk({ oid: oid, authToken: authToken, environmentUrl: environmentUrl });
var app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
app.use(cors());
app.post("/start-transaction", function (req, res) {
var amount = "0.01";
var config = {
transactionType: sdk.TransactionType.CreditSale,
method: "modal",
fields: [
{
id: "base_amount",
value: amount
},
{
id: "external_tran_id",
value: emergepay.getExternalTransactionId()
}
]
};
emergepay.startTransaction(config)
.then(function (transactionToken) {
res.send({
transactionToken: transactionToken
});
})
.catch(function (err) {
res.send(err.message);
});
});
console.log("listening on port 5555");
app.listen(5555);
//transactionType: "CreditSale"
//install the npm modules: 'npm install express cors emergepay-sdk
import * as express from "express";
import * as cors from "cors";
import {emergepaySdk, TransactionType} from "emergepay-sdk";
//Your private and secure creditials
const oid: string = "your_oid";
const authToken: string = "your_authToken";
const environmentUrl: string = "environment_url";
const emergepay: emergepaySdk = new emergepaySdk({oid, authToken, environmentUrl});
const app: any = express();
app.use(express.json());
app.use(express.urlencoded({extended:true}));
app.use(cors());
//The client side can hit this endpoint by issuing a POST request to
//localhost:5555/start-transaction
//base_amount and external_tran_id are required in the fields array.
app.post("/start-transaction", (req, res) => {
const amount = "0.01";
const config: any = {
transactionType: TransactionType.CreditSale,
method: "hostedFields",
fields: [
{
id : "base_amount",
value : amount
},
{
id : "external_tran_id",
value : emergepay.getExternalTransactionId()
}
]
};
emergepay.startTransaction(config)
.then(transactionToken => {
res.send({
transactionToken: transactionToken
});
})
.catch(err => {
res.send(err.message);
});
});
console.log("listening on port 5555");
app.listen(5555);
//transactionType: "CreditSale"
public async Task<object> StartTransactionAsync(StartTransactionModel config)
{
var response = new object();
//Use your private and secure creditials
const string OID = "your_oid";
const string AUTH_TOKEN = "your_authToken";
const string ENDPOINT_URL = "environment_url";
string url = $"{ENDPOINT_URL}/orgs/{OID}/transactions/start";
var contents = new
{
transactionData = new
{
transactionType = "CreditSale",
method = "hostedFields",
fields = new[]
{
new { id = "base_amount", value = config.Amount },
new { id = "external_tran_id", value = Guid.NewGuid().ToString() },
new { id = "billing_name", value = config.BillingName },
new { id = "billing_address", value = config.BillingAddress },
new { id = "billing_postal_code", value = config.BillingPostalCode },
new { id = "transaction_reference", value = config.TransactionReference },
new { id = "cashier_id", value = config.CashierId }
}
}
};
try
{
using (var client = new HttpClient())
{
var transactionJson = JsonConvert.SerializeObject(contents);
HttpRequestMessage request = new HttpRequestMessage(HttpMethod.Post, url);
request.Headers.Add("Authorization", $"Bearer {AUTH_TOKEN}");
request.Content = new StringContent(transactionJson, Encoding.UTF8, "application/json");
var httpResponse = await client.SendAsync(request);
var data = await httpResponse.Content.ReadAsStringAsync();
response = JsonConvert.DeserializeObject(data);
}
}
catch (Exception exc)
{
throw exc;
}
return response;
}
//transactionType: "CreditSale"
array(
'transactionType' => 'CreditSale',
'method' => 'hostedFields',
'fields' => array(
array('id' => 'base_amount', 'value' => '0.01'),
array('id' => 'external_tran_id', 'value' => GUID())
)
)
);
//Configure the request
$request = curl_init($url);
curl_setopt($request, CURLOPT_HEADER, false);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
curl_setopt($request, CURLOPT_HTTPHEADER, array('Content-Type: application/json', 'Authorization: Bearer ' . $authToken));
curl_setopt($request, CURLOPT_POST, true);
curl_setopt($request, CURLOPT_POSTFIELDS, json_encode($body));
//Issue the request and get the response
$response = curl_exec($request);
curl_close($request);
echo $response;
function GUID()
{
if (function_exists('com_create_guid') === true)
{
return trim(com_create_guid(), '{}');
}
return sprintf('%04X%04X-%04X-%04X-%04X-%04X%04X%04X', mt_rand(0, 65535), mt_rand(0, 65535), mt_rand(0, 65535), mt_rand(16384, 20479), mt_rand(32768, 49151), mt_rand(0, 65535), mt_rand(0, 65535), mt_rand(0, 65535));
}
?>
Gravity Dashboard
A finger on the pulse of your business.
Additional Features
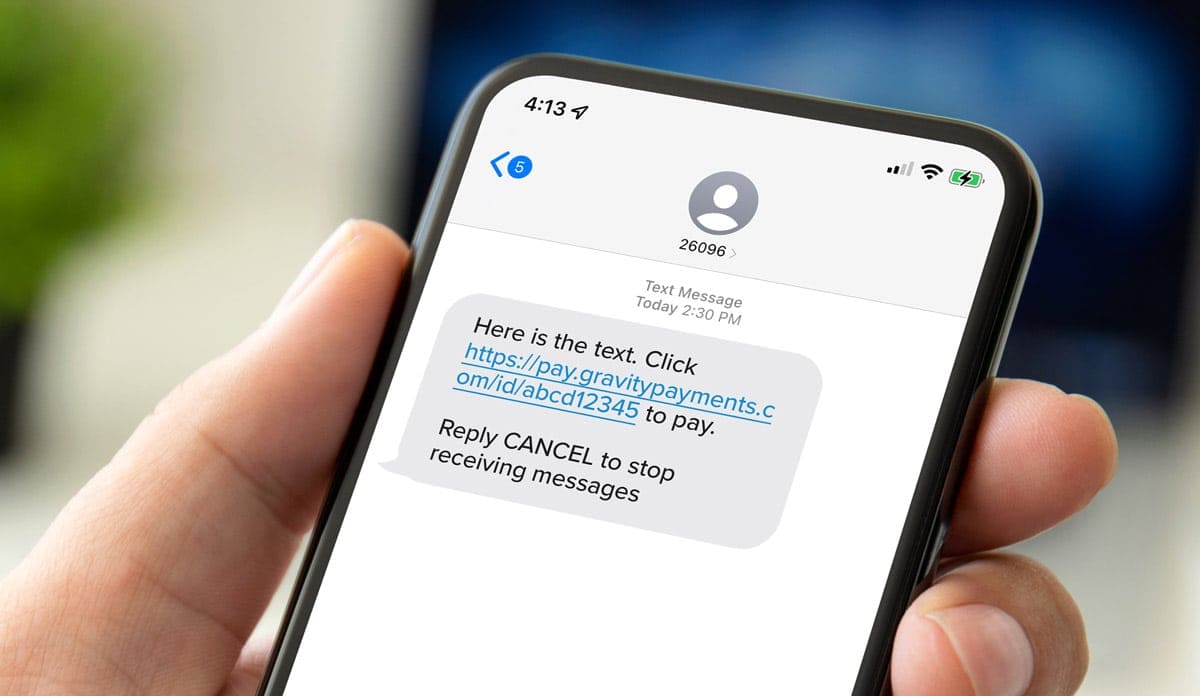
Text to Pay
SMS payment links for customer invoices.
Tokenized Transactions
Secure and unique strings of numbers that replace sensitive card data.
ACH Payments
Secure bank transfers without checks, credit cards, wire, or cash.
PCI Compliance & Data Security
Peace of mind comes with a high standard in data security.
Wireless/4G Devices
The latest and most functional devices on the market.
Secure Card Storage
Safe card storage for automated billing.
Local Payment Solutions
A cloud-alternative desktop app to communicate transaction information.
Want to work with Gravity or need development help?
Merchants
Tailored payments solutions, friendly financing, and 24/7 America-based support to help you grow your business with confidence.
Partners
Letās work together to broaden your reach, diversify your revenue, and better support main street small businesses.